Using Sign On PeopleCode to redirect Users to Specific pages
By Chris Malek | Mon, Oct 31, 2016
This article will give you some code examples and some ideas on how to use the sign on PeopleCode to redirect users to specific URLs or pages during both a successful and failure to login to PeopleSoft.
There are a couple of scenarios where the techniques described here would be useful.
Usage For Successful Sign On Scenarios
If you are already using some sign on PeopleCode to authenticate your users into PeopleSoft, you could add checks to redirect users to specific pages if something needs their attention.
- If the person has business expenses pending their approval, you can redirect them to the approval page.
- If the user has not entered some self-service payroll information, you can redirect them to that page.
- If the user has not validated their contact information you can redirect them to that page.
- If the user is late on their time sheet, you can redirect them to that page.
- If you have a certain set of users that may have limited PeopleSoft skill or training and they should really only be accessing a few pages. In this situation you may not want these users to have the burden to navigate in PeopleSoft. Your sign on PeopleCode could detect these users and redirect them to their desired start page.
I could imagine a configurable setup where you could configure the components that a user is redirected to based on some SQL that runs at the user level.
Unsuccessful sign on Scenarios
With sign on PeopleCode you can also redirect users to specific pages if the login failed. This can be useful in situations where an SSO system is sending users into PeopleSoft and some sort of error occurs.
- When the sign on PeopleCode runs to validate information passed from your SSO system and it cannot find a valid PeopleSoft user ID. In this scenario, you could redirect them to a central “error” page on your website that has information on where to get help. The default behavior of PeopleSoft would be to show an login page which could confuse users coming from an SSO system.
I think if you use standard PeopleSoft login, you would have to be careful with this technique or your code because you would not want to redirect a user to an error page if they just mis-typed their password.
System setup
We are going to setup a few limited examples of using sign on PeopleCode to redirect users.
(1) Create a sign on PeopleCode Users
First we need to create an OPRID that has zero access in the system. The account will have a password but no roles will be assigned. In order to get PeopleCode to run we have to have PeopleSoft setup with a “public user”. Your system may already have something like this setup.
In my case, I created an OPRID called “CHG_GUEST”. It has a password and zero roles.
(2) Configure Public Access in your Web Profile
Now we need to go to your web profile and setup public access using that “CHG_GUEST” user id.
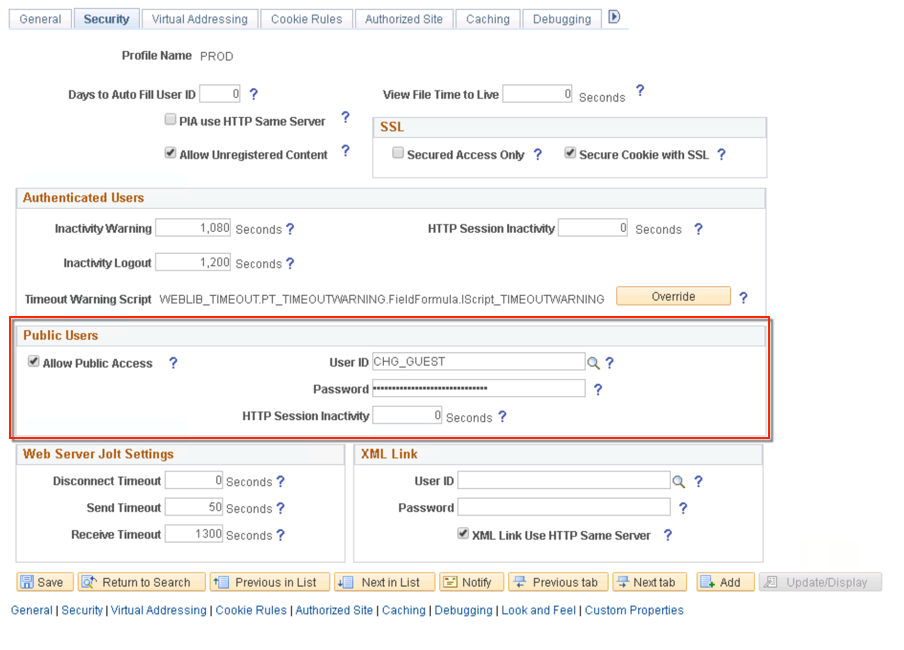
You will need to have your web server bounced after you make this change.
(3) Sign On PeopleCode
Now we will write some sign on PeopleCode called SignOnRedirectTest
in CHG_SIGN_ON.FUNCLIB.FieldDefault
. We then activate that sign on PeopleCode like this. (see sequence 10)
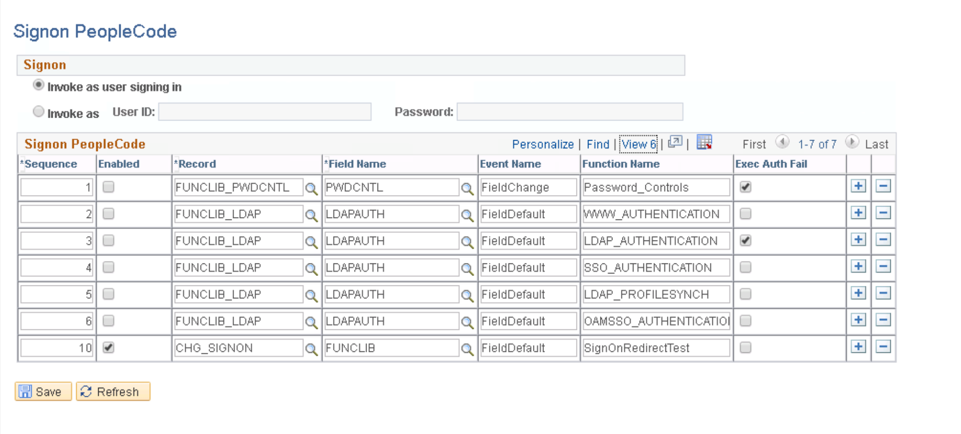
In this setup we have the “Exec Auth Fail” parameter set to be unchecked. This tells the system to only execute the PeopleCode if there was some successful login. This is true because we configured a public user that should always be authenticated. As you recall that public user has zero roles assigned. So even though the sign on PeopleCode will execute under that account no PeopleSoft page will ever be rendered for that “CHG_GUEST” user. This is a subtle but important point. There are other use cases where it would be appropriate to set the “Exec Auth FaiL” to be checked.
Here is our example code. This is obviously not production code.
Function signonRedirectTest()
Local boolean &bSimulateFailure = True;
If %PSAuthResult = True And
%signonUserId = "CHG_GUEST" Then
Local string &resultDocScript = "<script type='text/javascript'>window.location='{{REDIRECT_URL}}';</script>";
Local string &resultDoc;
If &bSimulateFailure Then
Local string &FailURL = "https://www.google.com/search?q=FAIL+WHALE";
&resultDoc = Substitute(&resultDocScript, "{{REDIRECT_URL}}", &FailURL);
SetAuthenticationResult( False, "", &resultDoc, False, 0);
Else
Local string &hardCodedUser = "PS"; /* Obviously do not do this in production */
Local string &SucessURL;
&SucessURL = GenerateComponentContentURL(%Portal, %Node, MenuName.SA_LEARNER_SERVICES, "GBL", Component.SSS_STUDENT_CENTER, "", "");
&resultDoc = Substitute(&resultDocScript, "{{REDIRECT_URL}}", &SucessURL);
SetAuthenticationResult( True, &hardCodedUser, &resultDoc, False, 0);
End-If;
End-If;
End-Function;
Unsuccessful Login
First we are going to look at the code branch of an unsuccessful login. Let’s go over the code sections step by step.
- First we have a parameter where we can simulate a login success or failure and control the branching of our test code.
Local boolean &bSimulateFailure = True;
- The next section is this:
If %PSAuthResult = True And
sign onUserId = "CHG_GUEST" Then
This section makes sure that this code only executes for the “CHG_GUEST” user. If some other piece of sign on PeopleCode authenticated the user then we do not run the code. We only want this code to run if no other sign on PeopleCode authenticated a user.
The next section for a failure is this section.
Local string &FailURL = "https://www.google.com/search?q=FAIL+WHALE";
&resultDoc = Substitute(&resultDocScript, "{{REDIRECT_URL}}", &FailURL);
SetAuthenticationResult( False, "", &resultDoc, False, 0);
- We define a failure URL. After this code runs the
&resultDoc
variable will contain this HTML / javascript code.- This will force the user’s browser to load a google page that shows search results for a Fail Whale.
"<script type='text/javascript'>window.location='{{REDIRECT_URL}}';</script>"
- We then call SetAuthenticationResult with an
authresult
parameter ofFalse
which basically says “Do NOT Authenticate the user”.- The function signature of SetAuthenticationResult is :
SetAuthenticationResult(AuthResult [, UserId] [,ResultDocument] [,PasswordExpired] [DaysLeftBeforeExpire]).
- The key piece to the puzzle is the
ResultDocument
document parameter which we will pass the&resultDoc
HTML/Javascript. The other parameters can be ignored. This causes the web server to load thesignonresultdoc.html
file merging in our value passed in theResultDocument
parameter.
- The function signature of SetAuthenticationResult is :
So the end user’s browser will end up going to the Fail Whale result if they have javascript enabled.
Successful Sign On
- Now let’s look at a successful sign on. We will change the code to mimic that by replacing this line of code.
Local boolean &bSimulateFailure = False;
- In this case, we have some hard coded user.
Local string &hardCodedUser = "PS"; /* Obviously do not do this in production */
- We then generate a URL that we want the user to go be redirected to at login. In this case, we are sending the user to the Student Center. You could easily have some logic here go to different URLs based on different parameters.
Local string &SucessURL;
&SucessURL = GenerateComponentContentURL(%Portal, %Node, MenuName.SA_LEARNER_SERVICES, "GBL", Component.SSS_STUDENT_CENTER, "", "");
&resultDoc = Substitute(&resultDocScript, "{{REDIRECT_URL}}", &SucessURL);
- In the call to SetAuthenticationResult, we pass parameters to the function that the user current user to be switched to the “PS” user and that they should be redirected to the student center.
SetAuthenticationResult( True, &hardCodedUser, &resultDoc, False, 0);
Article Categories
Chris Malek
Chris Malek is a PeopleTools® Technical Consultant with two decades of experience working on PeopleSoft enterprise software projects. He is available for consulting engagements.
About Chris Work with ChrisPeopleSoft Simple Web Services (SWS)
Introducing a small but powerful PeopleSoft bolt-on that makes web services very easy. If you have a SQL statement, you can turn that into a web service in PeopleSoft in a few minutes.
Integration Broker - The Missing Manual
I am in the process of writing a book called "Integration Broker - The Missing Manual" that you can read online.